5 Data Viz with ggplot2, themes
5.1 Built-in Themes
ggplot2
allows you to have fine control over all visual aspects of the plot. The default ggplot2
theme is theme_gray()
(or theme_grey()
will also work), but you can also choose theme_bw()
, which produces a more minimalist plot. You can set the base size of a plot as an argument.
z <- ggplot(mtcars, aes(x = wt, y = mpg, colour = factor(cyl))) +
geom_point(alpha = 0.6, size = 3, shape = 16) +
scale_y_continuous("Miles/(US) gallon",
limits = c(10, 40),
breaks = seq(10, 40, 10),
expand = c(0,0)) +
scale_x_continuous("Weight (lb/1000)",
limits = c(1,6),
breaks = 1:6,
expand = c(0,0)) +
scale_colour_brewer("Cylinders")
z
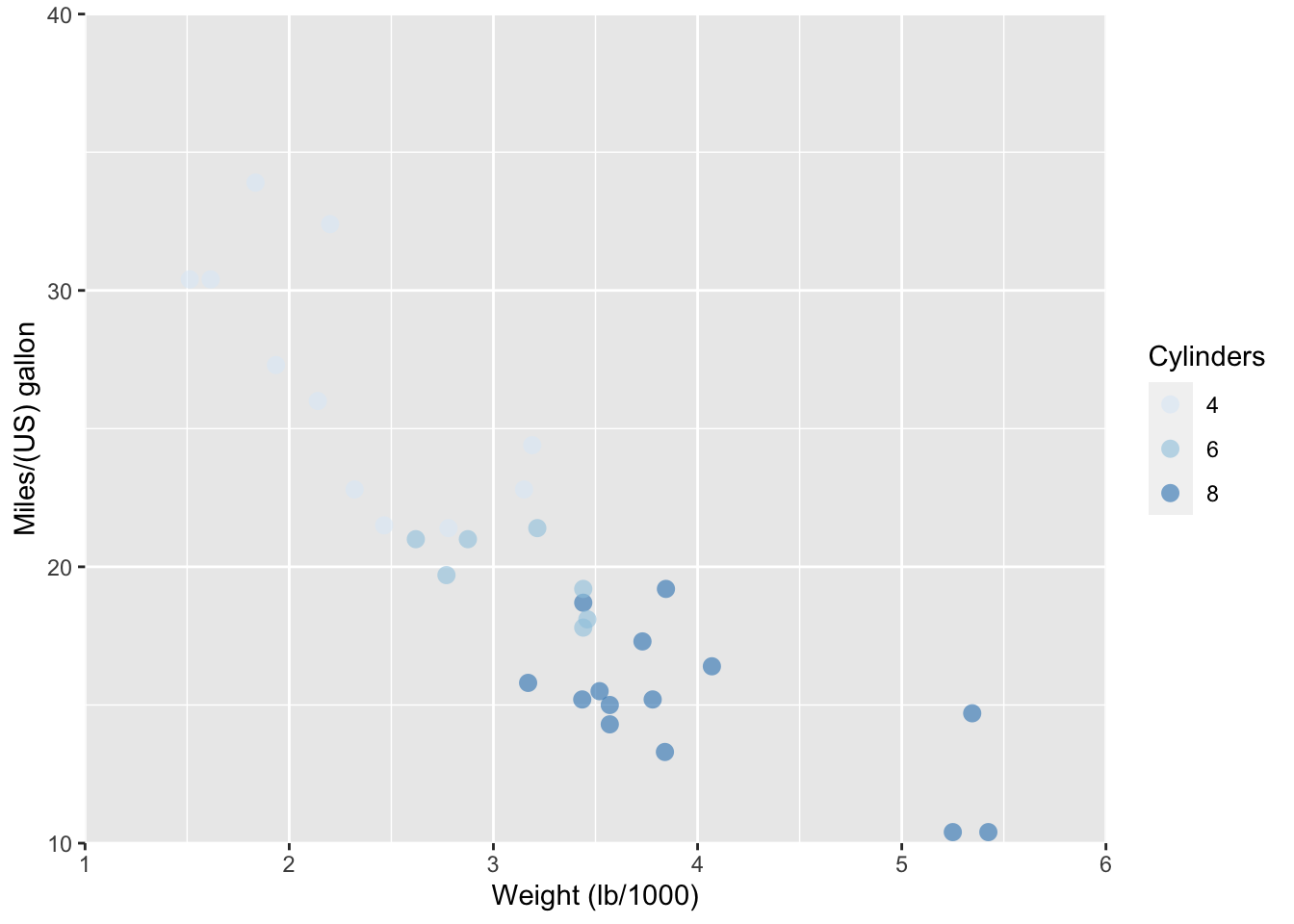
Figure 5.1: The ggplot2 default theme_gray() and magnified theme_bw().
# Black and White theme, with larger base size, shown right:
z + theme_classic(12)

5.2 Custom themes:
You can change individual aspects by modifying the default template, as shown below.
# Custom theme, shown right:
z + theme(rect = element_blank(),
axis.ticks = element_line(colour = "#A50F15"),
axis.text = element_text(colour = "#A50F15"))
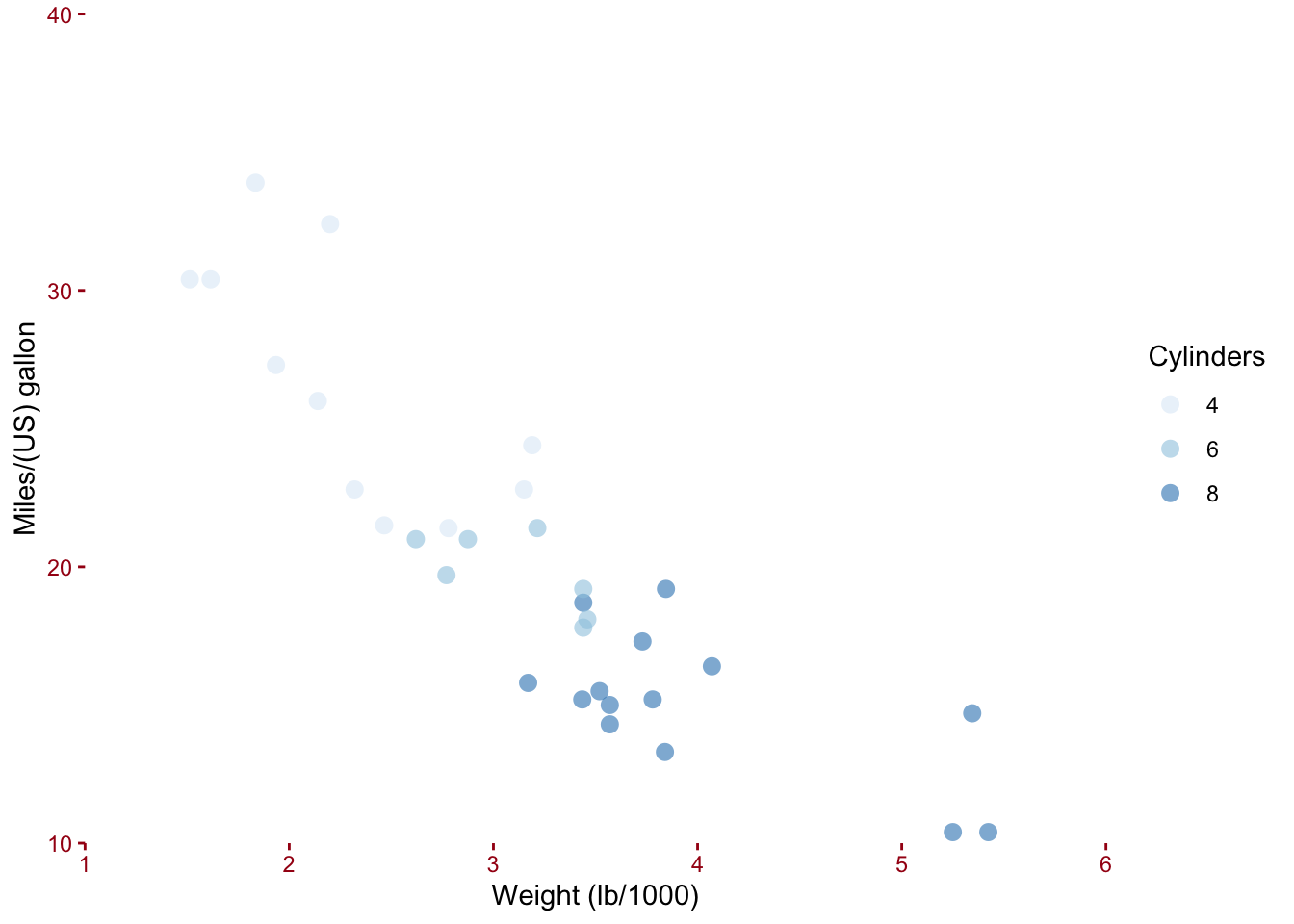
Figure 5.2: An example of updating an individual ggplot with custom theme settings using the theme()
function.
Arguments for the themes layer in ggplot2 v 3.2.1
:
Text, modify with element_text()
-
text
-
title
legend.title
plot.title
plot.subtitle
plot.caption
plot.tag
-
axis.title
-
axis.title.x
axis.title.x.top
axis.title.x.bottom
-
axis.title.y
axis.title.y.left
axis.title.y.right
-
-
axis.text
-
axis.text.x
axis.text.x.top
axis.text.x.bottom
-
axis.text.y
axis.text.y.left
axis.text.y.right
-
legend.text
-
strip.text
strip.text.x
strip.text.y
-
Lines, modify with element_line()
-
line
-
axis.ticks
-
axis.ticks.x
axis.ticks.x.top
axis.ticks.x.bottom
-
axis.ticks.y
axis.ticks.y.left
axis.ticks.y.right
-
-
axis.line
-
axis.line.x
axis.line.x.top
axis.line.x.bottom
-
axis.line.y
axis.line.y.left
axis.line.y.right
-
-
panel.grid
-
panel.grid.major
panel.grid.major.x
panel.grid.major.y
-
panel.grid.minor
panel.grid.minor.x
panel.grid.minor.y
-
-
Rect, modify with element_rect()
-
rect
legend.background
legend.key
panel.background
panel.border
plot.background
-
strip.background
strip.background.x
strip.background.y
Units, specify with unit()
-
axis.ticks.length
-
axis.ticks.length.x
axis.ticks.length.x.top
axis.ticks.length.x.bottom
-
axis.ticks.length.y
axis.ticks.length.y.left
axis.ticks.length.y.right
-
-
legend.spacing
legend.spacing.x
legend.spacing.y
-
legend.key.size
legend.key.height
legend.key.width
legend.box.spacing
-
panel.spacing
panel.spacing.x
panel.spacing.y
plot.margin
strip.switch.pad.grid
strip.switch.pad.wrap
Positioning and sizes
aspect.ratio
legend.margin
legend.text.align
legend.title.align
legend.position
legend.direction
legend.justification
-
legend.box
legend.box.just
legend.box.margin
legend.box.background
panel.ontop
plot.tag.position
strip.placement
When creating new themes from scratch:
-
complete
,TRUE
if this is a complete theme, e.g.theme_grey()
. Complete themes behave differently when added to a ggplot object. All elements will be set to inherit from blank elements. Defaults toFALSE
. -
validate
,TRUE
to run validate_element(), defaults toFALSE
.
It’s common to combine your custom theme with a built in theme:
# Custom theme, shown right:
z + theme_classic() +
theme(rect = element_blank(),
axis.ticks = element_line(colour = "#A50F15"),
axis.text = element_text(colour = "#A50F15"))

Which can also be saved as its own layer:
myTheme <- theme_classic() +
theme(rect = element_blank(),
axis.ticks = element_line(colour = "#A50F15"),
axis.text = element_text(colour = "#A50F15"))
z + myTheme

5.3 Updating the default theme:
# Save theme_gray() settings, and make an update:
original <- theme_update(panel.background = element_blank(),
legend.key = element_blank(),
axis.line = element_line(colour = "black"),
legend.position = c(.9, .9),
panel.grid.major = element_blank(),
panel.grid.minor = element_blank(),
axis.title.x = element_text(vjust = -0.3),
axis.text.x = element_text(colour = "black"),
axis.text.y = element_text(colour = "black")
)
# Plot with updated theme_gray() theme, shown left
z
# Reset original theme_gray() settings
theme_set(original)
# Plot with original theme_gray() settings, shown right
z